This problem 2 is “Rainbow Write a GraphicsProgram subclass that draws a rainbow”, the basics of the problem are “Starting at the top, the six arcs are red, orange, yellow, green, blue, and magenta, respectively; cyan makes a lovely colour for the sky. The top of the arc should not be off the screen. Each of the arcs in the rainbow should get clipped along the sides of the window,and not along the bottom. Your rainbow should be symmetric and nicely drawn, regardless of window size.”
The way that I thought about this, is to basically use the GOval to create a circle that is placed in the centre width and slowly go down on the Y coordinates, the size of the GOval is going to be twice the size of the screen itself, thus will meet the problem above. I setup a array of colours, that will then go through within a for loop to draw the GOval(circles) on the screen as below
Color colours[] = new Color[7]; colours[0] = Color.RED; colours[1] = Color.ORANGE; colours[2] = Color.YELLOW; colours[3] = Color.GREEN; colours[4] = Color.BLUE; colours[5] = Color.MAGENTA; colours[6] = Color.CYAN; for (int i =0; i < 7; i++) { GOval newCircle = new GOval(-getWidth()/2,10+(i*10), getWidth()*2, getHeight()*2); newCircle.setColor(colours[i]); newCircle.setFillColor(colours[i]); newCircle.setFilled(true); add(newCircle); } |
Here is my output of my Rainbow to the solution of the problem
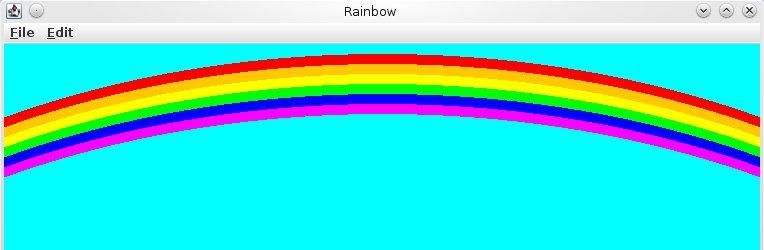
I have included the full source code in the zip file above., but here is the full source code for this problem.
/* * File: Rainbow.java * ------------------ * This program is a stub for the Rainbow problem, which displays * a rainbow by adding consecutively smaller circles to the canvas. */ import acm.graphics.*; import acm.program.*; import java.awt.*; public class Rainbow extends GraphicsProgram { public void run() { Color colours[] = new Color[7]; colours[0] = Color.RED; colours[1] = Color.ORANGE; colours[2] = Color.YELLOW; colours[3] = Color.GREEN; colours[4] = Color.BLUE; colours[5] = Color.MAGENTA; colours[6] = Color.CYAN; GRect background = new GRect(0,0,getWidth(), getHeight()); background.setColor(Color.CYAN); background.setFillColor(Color.CYAN); background.setFilled(true); add(background); for (int i =0; i < 7; i++) { GOval newCircle = new GOval(-getWidth()/2,10+(i*10), getWidth()*2, getHeight()*2); newCircle.setColor(colours[i]); newCircle.setFillColor(colours[i]); newCircle.setFilled(true); add(newCircle); } } } |
Completed as if a complete newbie to programming and knew only the methods/classes as shown in the videos and lecture notes. Don’t have the book, so I didn’t know if arrays were covered.