As from the UDP Client here is the UDP Server, it is similar to the TCP Server in that it makes connections with a remote client and sends and receives data, but once again this data is not checked to make sure that it has been received (the “fire and forget” setup again)
So to start with we need to create a server socket for the clients to connect to
DatagramSocket theSocket = new DatagramSocket(9999); |
since we now have a place for the client to connect to we now need to wait for a client connection request
// create a place for the client to send data too theRecievedPacket = new DatagramPacket(inBuffer, inBuffer.length); // wait for a client to request a connection theSocket.receive(theRecievedPacket); |
once a client has requested some data, we can start the process of setting up the response, the response starts of with getting there details, there IP address and also the port number that they wish to talk on ( image attached below of the wireshark request and different port number)
// get the client details clientAddress = theRecievedPacket.getAddress(); clientPort = theRecievedPacket.getPort(); |
and then just build up the response to send back and send it
String message = "Server - client sent : " + new String(theRecievedPacket.getData(),0, theRecievedPacket.getLength()); outBuffer = message.getBytes(); // send some data to the client theSendPacket = new DatagramPacket(outBuffer, outBuffer.length, clientAddress, clientPort); theSocket.send(theSendPacket); |
here is the full java code
import java.io.*; import java.net.*; public class UDPServer { DatagramSocket theSocket = null; int serverPort = 9999; public UDPServer() { try { // create the server UDP end point theSocket = new DatagramSocket(serverPort); System.out.println("UDP Socket (end point) created"); } catch (SocketException ExceSocket) { System.out.println("Socket creation error : "+ ExceSocket.getMessage()); } } public void clientRequest() { DatagramPacket theRecievedPacket; DatagramPacket theSendPacket; InetAddress clientAddress; int clientPort; byte[] outBuffer; byte[] inBuffer; // create some space for the text to send and recieve data outBuffer = new byte[500]; inBuffer = new byte[50]; try { // create a place for the client to send data too theRecievedPacket = new DatagramPacket(inBuffer, inBuffer.length); // wait for a client to request a connection theSocket.receive(theRecievedPacket); System.out.println("Client connected"); // get the client details clientAddress = theRecievedPacket.getAddress(); clientPort = theRecievedPacket.getPort(); String message = "Server - client sent : " + new String(theRecievedPacket.getData(),0, theRecievedPacket.getLength()); outBuffer = message.getBytes(); System.out.println("Client data sent ("+message+")"); // send some data to the client theSendPacket = new DatagramPacket(outBuffer, outBuffer.length, clientAddress, clientPort); theSocket.send(theSendPacket); } catch (IOException ExceIO) { System.out.println("Error with client request : "+ExceIO.getMessage()); } // close the server socket theSocket.close(); } public static void main(String[] args) { UDPServer theServer = new UDPServer(); theServer.clientRequest(); } } |
if you save that as UDPServer.java and then compile and run
javac UDPServer.java |
and then run the java UDPserver program, I have inserted the “— waiting for the client to connect” line because that is where the server will stop waiting, once the client as connected the rest of the output will be outputted.
java UDPServer UDP Socket (end point) created ---- waiting for the client to connect Client connected Client data sent (Server - client sent : genux) |
and then run the UDPClient to connect to the server
java UDPClient
Client socket created
Message sending is : genux
Client - server response : Server - client sent : genux |
Here is the image of a wireshark connection request over the UDP and with the clients port request number.
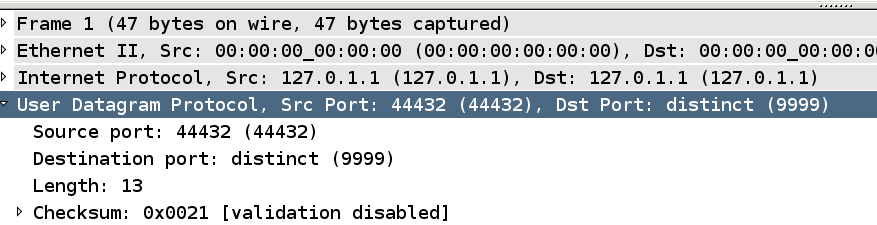